Here are the important Questions for Interviews on DSA to understand, if you are going for interview where DSA questions will be asked then you can read these questions and get answers. Get Important Interview questions on DSA (Data Structures & Algorithms) here…
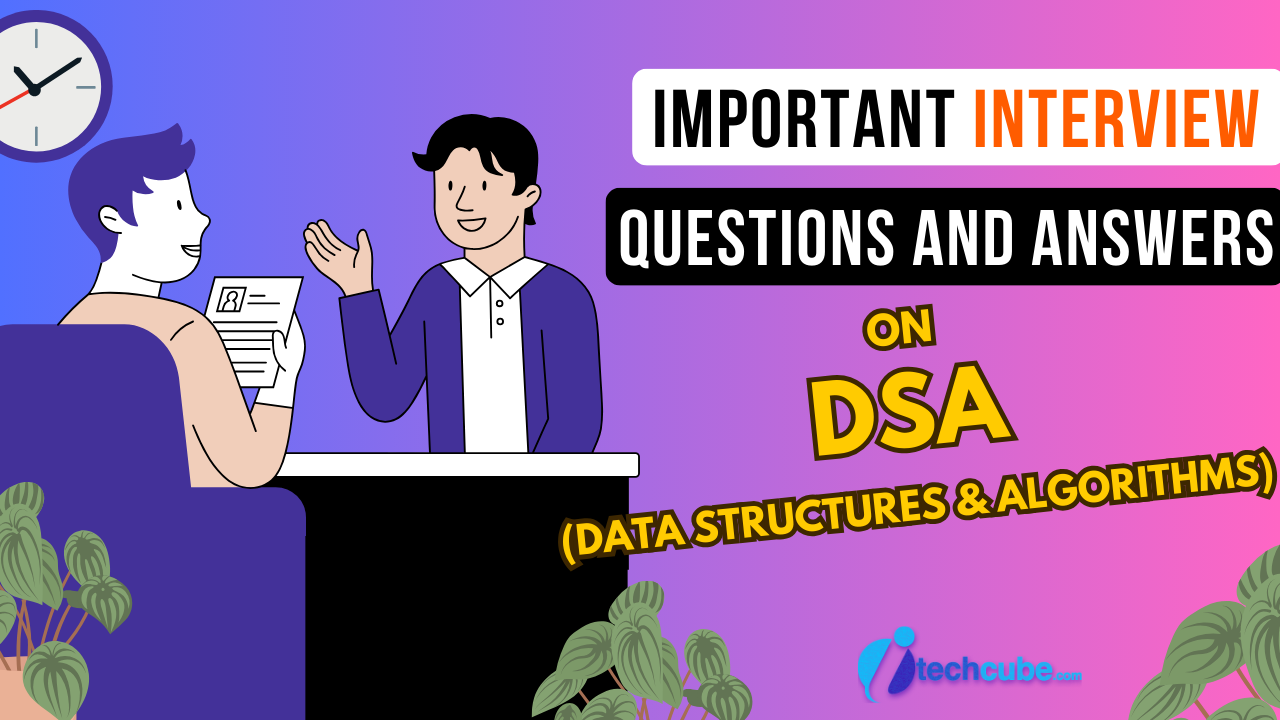
Interview Questions on DSA
What is DSA?
DSA stands for Data Structures and Algorithms. It’s the core of coding. Data Structures help store and organize data. Algorithms help solve problems using logic and steps.
Why is DSA important in coding interviews?
Because it shows how well you can think logically, solve problems, and write optimized code — something every company values.
What are the main types of Data Structures?
- Linear: Arrays, Linked Lists, Stacks, Queues
- Non-linear: Trees, Graphs
- Hash-based: Hash Tables, Hash Maps
- Others: Heaps, Tries, Sets
What is the difference between Stack and Queue?
- Stack uses Last In First Out (LIFO). Like a stack of plates.
- Queue uses First In First Out (FIFO). Like a queue at the ticket counter.
What is a Linked List?
A Linked List is a series of connected nodes. Each node holds data and a pointer to the next. Unlike arrays, the memory isn’t continuous.
What is the difference between Linear and Binary Search?
- Linear Search: Go through each element one by one. Time complexity O(n).
- Binary Search: Works on sorted arrays. Keep dividing the array. Time complexity O(log n).
What is Recursion?
Recursion is when a function calls itself. You must define a base case to stop it. Example: Calculating factorial or Fibonacci numbers.
What is the time complexity of operations in Array, Linked List, and HashMap?
- Access: Array – O(1), Linked List – O(n), HashMap – O(1)
- Insertion at end: Array – O(1), Linked List – O(1), HashMap – O(1)
- Deletion at start: Array – O(n), Linked List – O(1), HashMap – O(1)
- Search: Array – O(n), Linked List – O(n), HashMap – O(1)
What is Big O Notation? It represents the time/space complexity of an algorithm in the worst case.
- O(1): Constant time
- O(n): Linear time
- O(log n): Logarithmic time
- O(n^2): Quadratic time
What are some popular algorithms in interviews?
- Searching (Linear Search, Binary Search)
- Sorting (Bubble Sort, Merge Sort, Quick Sort)
- Recursion & Backtracking
- Tree Traversals (Inorder, Preorder, Postorder)
- Graph Algorithms (DFS, BFS)
- Dynamic Programming
- Two Pointer and Sliding Window Techniques